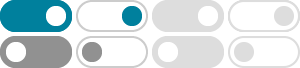
Difference between a LinkedList and a Binary Search Tree
Oct 26, 2015 · A linked list is a sequence of element where each element is linked to the next one, and in the case of a doubly linked list, the previous one. A binary search tree is something totally different. It has a root node, the root node has up to two child nodes, and each child node can have up to two child notes etc etc.
What is the best way to implement Tree : LinkedList - Array
Mar 10, 2014 · A linked list implementation would probably need to also store a reference to the position as to where their ancestors/children are, thus requiring an O(N) pass through the list to get the desired references. Search. Starting at the …
Implementing General Tree using the Linked List data structure
Jun 14, 2011 · Single_linked_list< SomeClassOrType > my_list; putThingsOnList( &my_list ); my_list.goToFirst(); while( !my_list.hasNext() ) { SomeClassOrType &o = children.getCurrent(); children.goToNext(); } The first part of your statement quoted above is correct: the * specifies a pointer type. The second part of your statement is where I disagree: the ...
LinkedList based tree in java - Stack Overflow
Nov 21, 2014 · sorry @fabian, I didnt give more details as i thought it will make it more complicated. Im trying to use the tree to solve an optimization problem using Branch&Bound algorithm. there's an upper and lower bound for the solution, the root is the optimum solution when starting, then we try to find a subset that can deliver a better solution and still satisfy the bounds, if a child can not satisfy ...
Convert linked list to tree in Java - Stack Overflow
Nov 8, 2014 · A linked list has an order n lookup time O(n) whereas a binary tree has a O(log(n)) lookup time. A linkedlist has constant time insert O(1) whereas a binary tree will have O(log(n)) insert. – Robert Bain
Linked List v.s. Binary Search Tree Insertion Time Complexity
Oct 13, 2021 · The insertion time of a Linked List is actually depends on where you are inserting and the types of Linked List. For example consider the following cases: You are using a single linked list and you are going to insert at the end / middle, you would have running time of O(n) to traverse the list till the end node or middle node.
When do you know when to use a TreeSet or LinkedList?
Sep 23, 2010 · By contrast a LinkedList is a List and therefore does allow duplicates and does preserve insertion order. On the performance side, TreeSet gives you O(logN) insertion and deletion, whereas LinkedList gives O(1) insertion at the beginning or end, and O(N) insertion at a selected position or deletion.
Should Binary Heap be a binary tree or linked list?
Feb 19, 2012 · A binary heap can be anything i.e. array, linked list, tree, etc. We just have to keep the right algorithm on how can you can access the data. For example, if you want to make it to the left child you can do this by 2N + 1(For starting index 0) where N is the parent index or the right child by 2N + 2. For the last element, you can initialise ...
Binary Trees, arrays vs linked - Stack Overflow
Mar 24, 2015 · It is also possible with a significant effort to implement the insert operation by maintaining the tree in a balanced shape. Simplifying a bit, you maintain a nearly-complete tree with storage size no more than 2n (where n is the current number of items in the tree). When a new item is inserted, you check where the appropriate array cell to ...
Traversing a tree using linked list structure - Stack Overflow
Sep 21, 2015 · I have a struct node which contains a next and a child. struct Node{ Node *parent; Node *next; Node *child; } The following picture shows the structure of the tree. How would I write a function...