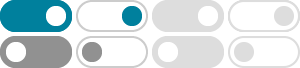
What does the ** maths operator do in Python? - Stack Overflow
From the Python 3 docs: The power operator has the same semantics as the built-in pow() function, when called with two arguments: it yields its left argument raised to the power of its right argument. The numeric arguments are first converted to a common type, and the result is of that type. It is equivalent to 2 16 = 65536, or pow(2, 16)
What does colon equal (:=) in Python mean? - Stack Overflow
Mar 21, 2023 · In Python this is simply =. To translate this pseudocode into Python you would need to know the data structures being referenced, and a bit more of the algorithm implementation. Some notes about psuedocode::= is the assignment operator or = in Python = is the equality operator or == in Python ; There are certain styles, and your mileage may vary:
Is there a "not equal" operator in Python? - Stack Overflow
Jun 16, 2012 · Python is dynamically, but strongly typed, and other statically typed languages would complain about comparing different types. There's also the else clause: # This will always print either "hi" or "no hi" unless something unforeseen happens. if hi == "hi": # The variable hi is being compared to the string "hi", strings are immutable in Python ...
What does the "at" (@) symbol do in Python? - Stack Overflow
Jun 17, 2011 · Functions, in Python, are first class objects - which means you can pass a function as an argument to another function, and return functions. Decorators do both of these things. If we stack decorators, the function, as defined, gets passed first to the decorator immediately above it, then the next, and so on.
What is Python's equivalent of && (logical-and) in an if-statement?
Mar 21, 2010 · There is no bitwise negation in Python (just the bitwise inverse operator ~ - but that is not equivalent to not). See also 6.6. Unary arithmetic and bitwise/binary operations and 6.7. Binary arithmetic operations. The logical operators (like in many other languages) have the advantage that these are short-circuited.
Exponentials in python: x**y vs math.pow (x, y) - Stack Overflow
Jan 7, 2014 · Difference between Python built-in pow and math pow for large integers. 1. Java Math.exp() and Python math ...
python - What is the purpose of the -m switch? - Stack Overflow
You must run python my_script.py from the directory where the file is located. Alternatively - python path/to/my_script.py. However, you can run python -m my_script (ie refer to the script by module name by omitting the .py) from anywhere, as long as Python can find it! Python searches as follows (not 100% sure about the order): Current directory
python - What does the caret (^) operator do? - Stack Overflow
Dec 14, 2021 · Side note, seeing as Python defines this as an xor operation and the method name has "xor" in it, I would consider it a poor design choice to make that method do something not related to xor like exponentiation. I think it's a good illustrative example of how it simply calls the __xor__ method, but to do that for real would be bad practice. –
What is :: (double colon) in Python when subscripting sequences?
Aug 10, 2010 · When slicing in Python the third parameter is the step. As others mentioned, see Extended Slices for a nice overview. With this knowledge, [::3] just means that you have not specified any start or end indices for your slice. Since you have specified a step, 3, this will take every third entry of something starting at the first index. For example:
python - Is there a difference between "==" and "is"? - Stack …
Since is for comparing objects and since in Python 3+ every variable such as string interpret as an object, let's see what happened in above paragraphs. In python there is id function that shows a unique constant of an object during its lifetime. This id is using in back-end of Python interpreter to compare two objects using is keyword.